Today I am going to show you another way to implement the example demonstrated in the last episode about command pattern.
Here is the UML diagram for command pattern and it will be implemented as usual in C#.
This time Command block is about to become an abstract class, and LightOnCommand is one of its concrete commands.
Let us take a look at Receiver block, which contains four public methods, including On, Off, Up and Down.
Receiver Portion:
In this example, the receiver plays a role as a light, which is responsible to execute the command that activated by the corresponding concrete commands.
For example, LightOnCommand has two public methods named Execute and Unexecute.
Light is turned on whenever Execute method is called, while to be turned off if Unexecute method is called.
Besides the light is instantiated and also passed to the construction of LightOnCommand at the beginning.
Like this: new LightOnCommand( light ), or new LightOffCommand( light ) and so on….
1: public class Light
2: {
3: public void On()
4: {
5: Console.WriteLine("Turn on the light.");
6: }
7: public void Off()
8: {
9: Console.WriteLine("Turn off the light.");
10: }
11: public void Up()
12: {
13: Console.WriteLine("Turn the light up.");
14: }
15: public void Down()
16: {
17: Console.WriteLine("Turn the light down.");
18: }
19: }
Command Portion:
1: public abstract class Command
2: {
3: protected Light light;
4: public Command(Light light)
5: {
6: this.light = light;
7: }
8: public abstract void Execute();
9: public abstract void Unexecute();
10: }
LightOnCommand Portion:
1: public class LightOnCommand : Command
2: {
3: public LightOnCommand(Light light) : base(light) { }
4:
5: public override void Execute()
6: {
7: light.On();
8: }
9:
10: public override void Unexecute()
11: {
12: light.Off();
13: }
14: }
LightOffCommand Portion:
1: public class LightOffCommand : Command
2: {
3: public LightOffCommand(Light light) : base(light) { }
4:
5: public override void Execute()
6: {
7: light.Off();
8: }
9:
10: public override void Unexecute()
11: {
12: light.On();
13: }
14: }
LightUpCommand Portion:
1: class LightUpCommand:Command
2: {
3: public LightUpCommand(Light light) : base(light) { }
4:
5: public override void Execute()
6: {
7: light.Up();
8: }
9:
10: public override void Unexecute()
11: {
12: light.Down();
13: }
14: }
LightDownCommand Portion:
1: class LightDownCommand:Command
2: {
3:
4: public LightDownCommand(Light light) : base(light) { }
5:
6: public override void Execute()
7: {
8: light.Down();
9: }
10:
11: public override void Unexecute()
12: {
13: light.Up();
14: }
15: }
Invoker Portion:
Here Invoker is responsible to collect all commands in a Ilist.
One additional function is added that every command can be removed or canceled if needed by calling CancelLastCommand().
1: class Invoker
2: {
3: IList<Command> command = new List<Command>();
4: public Invoker(Command cmd)
5: {
6: command.Add(cmd);
7: }
8: public void SetCommand(Command cmd)
9: {
10: command.Add(cmd);
11: }
12: public void Execute()
13: {
14: foreach(Command cmd in command)
15: {
16: cmd.Execute();
17: }
18: }
19: public void CancelLastCommand()
20: {
21: command.RemoveAt(command.Count - 1);
22:
23: }
24: }
Client Portion:
1: static void Main(string[] args)
2: {
3: Light light = new Light();
4: Command onCmd = new LightOnCommand(light);
5: Command offCmd = new LightOffCommand(light);
6: Command upCmd = new LightUpCommand(light);
7: Command downCmd = new LightDownCommand(light);
8: Invoker invoker = new Invoker(onCmd);
9: invoker.SetCommand(upCmd);
10: invoker.SetCommand(downCmd);
11: invoker.SetCommand(upCmd);
12: invoker.SetCommand(upCmd);
13: invoker.CancelLastCommand();
14: invoker.SetCommand(offCmd);
15: invoker.Execute();
16: Console.ReadKey();
17: }
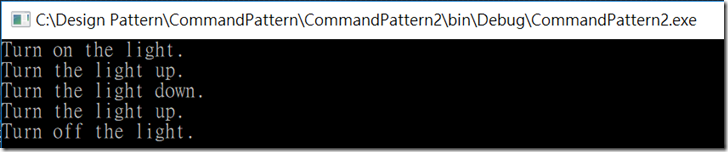